Help with JavaScript to Submit Form via Email!
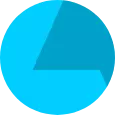
Copy link to clipboard
Copied
Using Adobe Acrobat Pro XI, I am working on a medical form form notifying our medical team of new patients. Upon completion of the form, I am trying to set it up to use a button to submit the saved PDF form and send it to our medical team in an email (we use MS Outlook across the organization). I've found multiple scripts that get me close to what I'm looking for, but I'm trying to accomplish this task with the following parameters:
Email To: "medical_team@outlook.com"
Subject Line: "Patient Notification Form - [LastName, FirstName Rank]"
Attach as: PDF
Body / Message: "Please find the attached Patient Notification Form for [LastName, FirstName Rank] attached."
Form Field Names:
- Last Name: Pull from form field "Patient_Last_Name"
- First Name: Pull from form field "Patient_First_Name"
- Rank: Pull from form field "Patient_Rank" Any help would be greatly appreciated!
Copy link to clipboard
Copied
You said that you are already using a script that got you close. What is missing? Please post the script and let us know what is not yet working correctly. It's much easier to fix an existing solution than to come up with one from scratch, so you will save both us and yourself some time by providing what you are already working with.
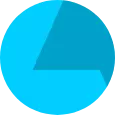
Copy link to clipboard
Copied
The requirements have actually changed slightly since my original post. The only difference that instead of rank, the team I am helping develop this form wants the Patient's Service (this is for the military). Basically, they are looking for the following:
Subject: Patient Notification Form - LastName, First Name (Service)
Body: Please find the attached Patient Notification Form for LastName, FirstName (Service).
Form Field Names:
LastName = Patient_Last_Name
FirstName = Patient_First_Name
Service = Patient_Service
The following script works in that it opens an email dialogue with the form attached:
var cToAddr = "medicalteam@mil"
var cSubLine = "Patient Notification Form"
var cBody = "Please find the attached Patient Notification Form"
this.mailDoc({bUI: true, cTo: cToAddr, cSubject: cSubLine, cMsg: cBody});
When I attempt to add the form field data, that is where I run into problems. Here is one of the scripts used most recently. Adobe accepts the script and lets me save it without producing any errors, but when the button is clicked, nothing happens.
var cToAddr = "medicalteam@mil"
var cSubLine = "Patient Notification Form - " + this.getField(Patient_Last_Name) + ", " + this.getField(Patient_First_Name) + " " + this.getField((Patient_Service))
var cBody = "Please find the attached Patient Notification Form for " + this.getField(Patient_Last_Name) + ", " + this.getField(Patient_First_Name) + " " + this.getField((Patient_Service))
this.mailDoc({bUI: true, cTo: cToAddr, cSubject: cSubLine, cMsg: cBody});
Copy link to clipboard
Copied
When you run the script, you are getting an error message on the console:
ReferenceError: Patient_Last_Name is not defined
2:Field:Mouse Up
The reason for this is that the Doc.getField() method expects a string as it's parameter (see here for more information: Acrobat DC SDK Documentation ) - you are however only providing an undefined variable (at least that's what the interpreter sees). In addition to this, just getting the field is not sufficient to get it's value, you need to access the field's "value" property. I've modified your code so that it should work:
var cToAddr = "medicalteam@mil";
var cSubLine = "Patient Notification Form - " + this.getField("Patient_Last_Name").value + ", " +
this.getField("Patient_First_Name").value + " " + this.getField("Patient_Service").value;
var cBody = "Please find the attached Patient Notification Form for " + this.getField("Patient_Last_Name").value + ", " +
this.getField("Patient_First_Name").value + " " + this.getField("Patient_Service").value;
this.mailDoc({
bUI: true,
cTo: cToAddr,
cSubject: cSubLine,
cMsg: cBody
});
In general, whenever you are not getting what you are expecting, the first step should be to check the JavaScript console for errors. Use Ctrl-J or Cmd-J to display the console window.
Copy link to clipboard
Copied
When using the getField method when successful the field object for the named field is returned but if the requested named field is not found then the return value of "null". One can test for this value and then appropriately handle the processing exception.
A possible script:
// could be a document level script available to all actions;
function GetField(oDoc, cName, bErrorMsg)
{
// return named field field object or null with optional error message;
if(typeof bErrorMsg == "undefined") var bErrorMsg = false;
// get named field object;
var oField = oDoc.getField(cName);
// check for error or not found;
if(oField == null)
{
// put error message in JavaScrpt Console;
console.println("Error accessing field " + cName);
// check for optional error message alert;
if(bErrorMsg)
{
app.alert("Error accessing field " + cName, 1, 0);
} // end if error message to be displayed
} // end return of null object;
// return requested object or null for failure;
return oField;
} // end GetField function;
// end document level funcitons;
// get field objects for email;
var oPatientFirstName = GetField(this, "Patient_First_Name", true);
var oPatientLastName = GetField(this, "Patient_Last_Name", true);
var oPatientService = GetField(this, "Patient_Service", true);
// process if fields not null; // could add test for non-empty fields;
if(oPatientFirstName != null && oPatientLastName != null && oPatientService != null)
{
var cToAddr = "medicalteam@mil";
var cSubLine = "Patient Notification Form - " + oPatientLastName.value + ", " +
oPatientFirstName.value + " " + oPatientService.value;
var cBody = "Please find the attached Patient Notification Form for " + oPatientLastName.value + ", " +
oPatientFirstName.value + " " + oPatientService.value;
this.mailDoc({
bUI: true,
cTo: cToAddr,
cSubject: cSubLine,
cMsg: cBody
});
} // end field values not null;
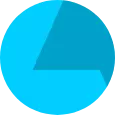
Copy link to clipboard
Copied
We're getting closer as the Outlook dialogue box opens now, but it's still not pulling the data from the form and including it in the subject/message as desired. Below is a copy of the script (copied directly from what you provided before) and an image of the output in the message dialogue box.
I really appreciate the assistance with this! I am definitely a newbie to Java and the link you provided was informative. I am, however, having trouble parsing through that information. I'm only speculating here, but my guess is the script properly reverences the data as desired, but does not populate it into the message dialogue... Could be wrong, just trying to learn as I go.
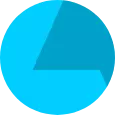
Copy link to clipboard
Copied
Additionally, below is the output from the Java Debugger
Copy link to clipboard
Copied
That looks like you've used gkaiseril's script. Have you tried mine, which is much less complex (but does not catch any errors with non-existing fields). BTW: This is JavaScript, not Java - very similar name, but completely different programming environment. This is important when you try to search for information. Whatever you find for Java will not be valid in the JavaScript environment.
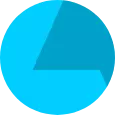
Copy link to clipboard
Copied
I used yours actually. I copied your script directly and just removed the line numbers and kept each line of script on the same line. Other than that it's identical.
I just tried to copy and paste it again and got the same result.
I actually had a difficult time understanding gkaiseril's script
Copy link to clipboard
Copied
The error message you posted refers to oPatientLastName, which is not in your original script or in my modified script. What errors are you getting when you just use my script?
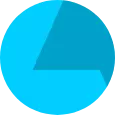
Copy link to clipboard
Copied
Ahh, my apologies, must have attached the wrong screenshot. With you're script I don't actually get an error. Below is the Script, Debugger Output and the resulting email dialogue output.
[ Mod: removed email addresses in screenshots ]
Copy link to clipboard
Copied
The second screenshot is useless since we can't see the full error message. Please post both the code as the error message as text, not as an image!
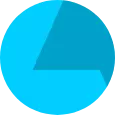
Copy link to clipboard
Copied
There wasn't anything above it when I first engaged the Debugger. I also can't copy and paste text into my web browser because the "Drag and Drop or Copy and Paste" is disabled by group policy and cannot be adjusted, otherwise I would have from the beginning.
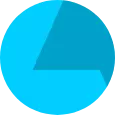
Copy link to clipboard
Copied
Not sure why it produced no errors the first time, but here is the output after re-opening the document and running the debugger. I haven't changed anything since my first reply. The output didn't fit in the debugger window without having to scroll and I can't copy and paste to my web browser. Below is the output copied to a Notepad file
Copy link to clipboard
Copied
This is the same type of error message you got in the beginning (or actually the error I got when I used your script). Are you sure that the correct script is associated with this button? Do you have multiple submit buttons, and you only changed the script for one?
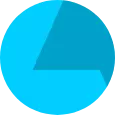
Copy link to clipboard
Copied
Yes, 100% certain. For kicks and giggles I even re-copied your script and pasted it back into the JavaScript console, removed the numbers again, and it produced exactly the same result
Copy link to clipboard
Copied
You can reply to a comment here via email, you do not have to use the web browser to write a reply. If it's easier for you to reply by email, and if that allows you to copy&paste, then please do so. Just make sure that you remove your email signature and any quoted content from the post you are replying to (that just clutters up the discussion and makes your reply harder to read).
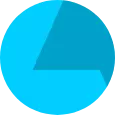
Copy link to clipboard
Copied
How does one go about replying via email? I see that I can hover over your avatar and select "Send Message", but it just opens another message dialogue in my browser which doesn't give me a workaround.
Copy link to clipboard
Copied
I assume you get an email notification when a new reply is posted. Just use the reply function when you read that message, remove anything that's already in the email editor (the quoted message you are replying to) and type your reply. You should be able to copy & paste (unless your IT department locked that down too).
Copy link to clipboard
Copied
Take a look at this sample PDF: It contains the three fields you are using for your script, and a submit button with my script from above.
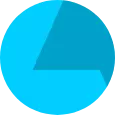
Copy link to clipboard
Copied
CLASSIFICATION: UNCLASSIFIED
Thanks for the advice so far! I really do appreciate it.
Below is the current JavaScript:
var cToAddr = "medicalteam@mil";
var cSubLine = "Patient Notification Form - " + this.getField("Patient_Last_Name").value + ", " + this.getField("Patient_First_Name").value + " " + this.getField("Patient_Service").value;
var cBody = "Please find the attached Patient Notification Form for " + this.getField("Patient_Last_Name").value + ", " + this.getField("Patient_First_Name").value + " " + this.getField("Patient_Service").value;
this.mailDoc();
And here is the JavaScript Debugger Output:
Acrobat EScript Built-in Functions Version 11.0
Acrobat SOAP 11.0
ReferenceError: Patient_Last_Name is not defined
2:Field:Mouse Up
ReferenceError: Patient_Last_Name is not defined
2:Field:Mouse Up
ReferenceError: Patient_Last_Name is not defined
2:Field:Mouse Up
SyntaxError: missing ; before statement
5:
SyntaxError: missing ; before statement
5:
SyntaxError: missing ; before statement
6:
SyntaxError: missing ; before statement
6:
SyntaxError: missing variable name
2:
SyntaxError: missing ; before statement
6:
GeneralError: Operation failed.
Doc.mailDoc:9:Field Submit_PNF:Mouse Up
CLASSIFICATION: UNCLASSIFIED
Copy link to clipboard
Copied
The last line just calls this.mailDoc() without any arguments, so your
"To", "Subject" and "Body" information is not getting used.
There is nothing in these lines that could generate the error message you
are seeing. You have some strange line breaks in the quoted script, I
assume that you are not breaking within a string, and that this is just due
to how the mail application formatted the code.
In Acrobat, you can get a list of all JavaScript that is in a document, you
do this via the "All JavaScripts" function in Tools>JavaScript. I would
pull that up and see if you have any more instances of referencing the
field names without the quotes around them.
Copy link to clipboard
Copied
There's clearly a scroll-bar there and it's at the bottom position, which means there's more text at the top.
And what kind of a ridiculous security policy is it that allows one to copy and paste images but not text? This is what's called "fake security"...
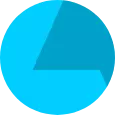
Copy link to clipboard
Copied
I didn't copy and paste the image, I used the insert function organic to the post reply console.
Copy link to clipboard
Copied
You need to scroll up in the Javascript console: We are seeing only the last line, which indicates where an error happened, but not what the error actually is.


-
- 1
- 2