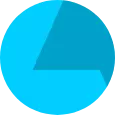
Copy link to clipboard
Copied
I am building a form for a small medical office. The provider wants a dialog box where he can check one or more of a few very common items. The form is being built on top of an existing document that has space limitations and cannot be modified. I have found tutorials for dialog boxes that ask for some user input, but nothing with multiple check boxes that allow me to pass the data back to my main form. I know this will involve some scripting, and I'm not afraid of that, but I am still learning javascript. I also have a budget of....nothing additional except my time, so joining a website isn't an option. I'm not asking for anyone to do this for me, just nudge me in the right direction that I can figure it out.
Thank you,
CalisMoonelf
1 Correct answer
The following modifications to the script should help:
...//Acrobat JavaScript Dialog
//Created by DialogDesigner from WindJack Solutions
var FormRouting =
{
result:"cancel",
DoDialog: function(){return app.execDialog(this);},
bChk1:false,
bChk2:false,
bChk3:false,
bChk4:false,
bChk5:false,
bChk6:false,
initialize: function(dialog)
{
var dlgInit =
{
"Chk1": this.bChk1,
"Chk2": this.bChk2,
"Chk3": this.bChk3,
Copy link to clipboard
Copied
Creating JavaScript Dialogs in Acrobat is non-trivial even for experienced programmers. If you're still learning JavaScript, don't jump right into the dialog code, start with something more simple so that you understand how to get and set values for fields. Also, read up on JSON, that's how the dialogs are defined. Finally, there are examples in the Acrobat JavaScript Documentation that show how to construct an dialog, look at those.
That said, it's absolutely worth joining pdfscripting.com if you're going to be attempting this as a novice. Skip Starbucks for a few days and you'll have the budget for the membership.
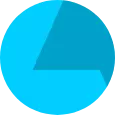
Copy link to clipboard
Copied
Joel_Geraci wrote
Skip Starbucks for a few days and you'll have the budget for the membership.
Starbucks must be a lot more expensive than I remember it from years ago. Membership is more than the weekly grocery budget for my family. LOL
Copy link to clipboard
Copied
Here's a link to a sample document that might help: http://www.windjack.com/resources/Examples/DialogUses.pdf
The last button (Control Document Processing) demonstrates a custom dialog with a number of check boxes.
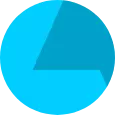
Copy link to clipboard
Copied
I have played with that particular file for hours, and have been unable to figure out how to pass the results back to my initial document. I learned how to add boxes, change the names etc, but passing them out has eluded me.
Copy link to clipboard
Copied
Generally speaking there are two ways of doing it:
- Define your variable before the definition of the dialog and then apply the values to them directly in the dialog's commit function, or
- Define your variables within the dialog itself as properties of the dialog (this.myVar1, this.myVar2, etc.) and then access them from outside the dialog (myDialog1.myVar1, myDialog1.myVar2).
I prefer the former, but both should work.
Copy link to clipboard
Copied
You do this by creating properties in the dialog object:
var myDialog = {
variable1: "",
variable2: true,
variable3: 34,
initialize: ...
Just keep in mind that you have to reference these properties using the 'this." syntax (e.g. this.variable1 = 23; ). Once you return from displaying the dialog object, you can then get the value of these properties using e.g. myDialog.variable1
Look at the script for the last button, it does actually demonstrate how data is passed from the dialog back to the script displaying the dialog.
Copy link to clipboard
Copied
Also, note that that sample has a few problems with the sample code. There are 6 check boxes (3 with the same item_id), but the code only deals with 4 of them, so don't let that get in the way of understanding it.
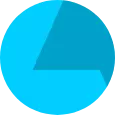
Copy link to clipboard
Copied
George_Johnson wrote
Also, note that that sample has a few problems with the sample code. There are 6 check boxes (3 with the same item_id), but the code only deals with 4 of them, so don't let that get in the way of understanding it.
That actually is the biggest part of what confused me. It looked to me that somehow the script passed all data collectively out and I couldn't for the life of me understand why. This comment probably has helped me more than any. Thank you!
Copy link to clipboard
Copied
The following modifications to the script should help:
//Acrobat JavaScript Dialog
//Created by DialogDesigner from WindJack Solutions
var FormRouting =
{
result:"cancel",
DoDialog: function(){return app.execDialog(this);},
bChk1:false,
bChk2:false,
bChk3:false,
bChk4:false,
bChk5:false,
bChk6:false,
initialize: function(dialog)
{
var dlgInit =
{
"Chk1": this.bChk1,
"Chk2": this.bChk2,
"Chk3": this.bChk3,
"Chk4": this.bChk4,
"Chk5": this.bChk5,
"Chk6": this.bChk6
};
dialog.load(dlgInit);
},
commit: function(dialog)
{
var oRslt = dialog.store();
this.bChk1 = oRslt["Chk1"];
this.bChk2 = oRslt["Chk2"];
this.bChk3 = oRslt["Chk3"];
this.bChk4 = oRslt["Chk4"];
this.bChk5 = oRslt["Chk5"];
this.bChk6 = oRslt["Chk6"];
},
description:
{
name: "Form Routing",
elements:
[
{
type: "view",
elements:
[
{
type: "view",
char_height: 10,
elements:
[
{
type: "static_text",
item_id: "stat",
name: "Route Form To:",
char_width: 15,
alignment: "align_fill",
font: "dialog",
},
{
type: "view",
char_width: 8,
char_height: 8,
align_children: "align_top",
elements:
[
{
type: "view",
char_width: 8,
char_height: 8,
elements:
[
{
type: "check_box",
item_id: "Chk1",
name: "Marketing",
},
{
type: "check_box",
item_id: "Chk2",
name: "Sales",
},
{
type: "check_box",
item_id: "Chk3",
name: "Accounting",
},
]
},
{
type: "view",
char_width: 8,
char_height: 8,
elements:
[
{
type: "check_box",
item_id: "Chk4",
name: "Engineering",
},
{
type: "check_box",
item_id: "Chk5",
name: "Division HQ",
},
{
type: "check_box",
item_id: "Chk6",
name: "Corporate",
},
]
},
]
},
]
},
{
type: "ok_cancel",
},
]
},
]
}
};
// Example Code
FormRouting.bChk1 = false;
FormRouting.bChk2 = false;
FormRouting.bChk3 = false;
FormRouting.bChk4 = false;
FormRouting.bChk5 = false;
FormRouting.bChk6 = false;
if("ok" == FormRouting.DoDialog())
{
console.println("Chk1:" + FormRouting.bChk1);
console.println("Chk2:" + FormRouting.bChk2);
console.println("Chk3:" + FormRouting.bChk3);
console.println("Chk4:" + FormRouting.bChk4);
console.println("Chk5:" + FormRouting.bChk5);
console.println("Chk6:" + FormRouting.bChk6);
}
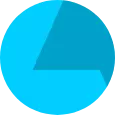
Copy link to clipboard
Copied
George_Johnson wrote
The following modifications to the script should help:
Wow! Above and beyond. Thank you for your help.
Copy link to clipboard
Copied
GM, I hope all is well. I wanted to reach out to first say thank you! for posting this example code. I was able to mimic its format to do what I wanted. (Sorry, I'm a newbie to javascript, much more comfortable with Python.) However, I am running into issues with tracking and capturing whether or not the checkbox is checked. My code works and tracks the first 9 check box responses, but after that each 'Text' box is labeled 'Undefined' even though I've defined them within the dialog box. (my screen shot only shows one 'Undefined' box as I've deleted the others since they are not working correctly) I've attached my code, the pop up menu screen shot, along with the results captured screen shot for reference. Any assistance with why my checkboxes aren't being captured is greatly appreciated. Thanks!
Copy link to clipboard
Copied
The id proeprty of each object in the dialog must be exactly 4 characters, no more and no less.
So it works up to "Chk9", but not with "Chk10" or higher.
Copy link to clipboard
Copied
Thank you! Much appreciated.
Copy link to clipboard
Copied
Again thanks for your assistance, .... another follow up if u don't mind. Can you point me in the right direction? I'm trying to get my text fields mentioned above to show default values of "N/A" when my form is opened, -- before its corresponding pop up menu is opened and check boxes are selected or not, which when the pop up is closed the text values will then display the coded "True" or "False" values.
Ive tried setting the default values property to "N/A" and used the "this.calculateNow()" method but to no avail. I've even used the ".defaultValue = true" as well as the "this.SetFormFieldData()" methods but nothing seems to work.
Any assistance is greatly appreciated, thanks.
Copy link to clipboard
Copied
If "N/A" is the default value of the field then you can use this:
this.resetForm(["FieldName"]);
Or more simply:
this.getField("FieldName").value = "N/A";
Copy link to clipboard
Copied
Thanks again for all your help. Would you mind educating me as I'm new to JavaScript... Note that all my text fields in question allow the user to custom edit the value.
I currently have script that when a drop down value is selected, it populates a select group of the aforementioned text fields with predetermined values. However when the value 'Custom -*' is selected from the drop down, the user input values aren't being stored in the aforementioned text fields once a second value is entered.
Ex. When user inputs a value of -18.0 in "Text1" the value is stored. But as soon as they enter a value in "Text2", "Text2" has its value stored and "Text1's" value disappears. Any idea as to why this is happening? Thanks.

