Copy link to clipboard
Copied
Hi all,
I'm trying to work with google api and javascript and show multiple location markers
I grabbed this code to start:
My goal is to get my address from my database and replace the hard coded addresses with my variable locations rather than theirs...
below might seem a bit odd but is example of my fail....
- I'm getting an error when I try to replace the harcoded text with my variable (with the same text)
---> this will cause error = geocode of undefined failed:OVER_QUERY_LIMIT
i think it's because it's a string not an array / object?
Q: how can I get this working and convert back as an array?
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>multi 1 Document</title>
<link rel="stylesheet" href="https://code.jquery.com/mobile/1.4.5/jquery.mobile.structure-1.4.5.min.css" />
<script src="https://code.jquery.com/jquery-1.11.1.min.js"></script>
<script src="https://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.js"></script>
<script type="text/javascript"
src=
"https://maps.googleapis.com/maps/api/js?v=3.exp&sensor=false">
</script>
<style type="text/css">
html, body, #map_canvas {
height: 500px;
width: 500px;
margin: 0px;
padding: 0px
}
</style>
</head>
<body>
<script>
// try to replace this working var with my version of same - makes error
var locations = [
['Location 1 Name', 'New York, NY', 'Location 1 URL'],
['Location 2 Name', 'Newark, NJ', 'Location 2 URL'],
['Location 3 Name', 'Philadelphia, PA', 'Location 3 URL']
];
// my attempt (really comes from database addresses - this simple for now)
var locations =
"[['Location 1 Name','New York, NY','Location 1 URL'], ['Location 2 Name','Newark, NJ','Location 2 URL'], ['Location 3 Name','Philadelphia, PA','Location 3 URL']]";
localStorage.setItem("tryit", locations);
// this will cause error = geocode of undefined failed:OVER_QUERY_LIMIT
// i think it's because it's a string not an array / object?
//Q: how can I get this working and convert back as an array?
var locations = localStorage.getItem("tryit");
var geocoder;
var map;
var bounds = new google.maps.LatLngBounds();
function initialize() {
map = new google.maps.Map(
document.getElementById("map_canvas"), {
center: new google.maps.LatLng(37.4419, -122.1419),
//center: new google.maps.LatLng(34.1623449, -118.3995877),
zoom: 13,
mapTypeId: google.maps.MapTypeId.ROADMAP
});
geocoder = new google.maps.Geocoder();
for (i = 0; i < locations.length; i++) {
geocodeAddress(locations, i);
}
}
google.maps.event.addDomListener(window, "load", initialize);
function geocodeAddress(locations, i) {
var title = locations[0];
var address = locations[1];
var url = locations[2];
geocoder.geocode({
'address': locations[1]
},
function (results, status) {
if (status == google.maps.GeocoderStatus.OK) {
var marker = new google.maps.Marker({
icon: 'http://maps.google.com/mapfiles/ms/icons/blue.png',
map: map,
position: results[0].geometry.location,
title: title,
animation: google.maps.Animation.DROP,
address: address,
url: url
})
infoWindow(marker, map, title, address, url);
bounds.extend(marker.getPosition());
map.fitBounds(bounds);
} else {
alert("geocode of " + address + " failed:" + status);
}
});
}
function infoWindow(marker, map, title, address, url) {
google.maps.event.addListener(marker, 'click', function () {
var html = "<div><h3>" + title + "</h3><p>" + address + "<br></div><a href='" + url + "'>View location</a></p></div>";
iw = new google.maps.InfoWindow({
content: html,
maxWidth: 350
});
iw.open(map, marker);
});
}
function createMarker(results) {
var marker = new google.maps.Marker({
icon: 'http://maps.google.com/mapfiles/ms/icons/blue.png',
map: map,
position: results[0].geometry.location,
title: title,
animation: google.maps.Animation.DROP,
address: address,
url: url
})
bounds.extend(marker.getPosition());
map.fitBounds(bounds);
infoWindow(marker, map, title, address, url);
return marker;
}
</script>
<div id="map_canvas" style="width:750px; height:450px; border: 2px solid #3872ac;"></div>
</body>
</html>
1 Correct answer

The issue is because your attempt of the var locations is actually a string, not an array that is needed to process a geolocation. That's why you get 150 thousand alert messages because it's trying to loop through each array item and process a geolocation, but since your var is a string it's looping through the length of the string, which is like 150 thousand characters. The solution is to build an array from your DB instead of building a string. Basically when you query your DB create an empty
...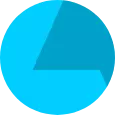
Copy link to clipboard
Copied
The issue is because your attempt of the var locations is actually a string, not an array that is needed to process a geolocation. That's why you get 150 thousand alert messages because it's trying to loop through each array item and process a geolocation, but since your var is a string it's looping through the length of the string, which is like 150 thousand characters. The solution is to build an array from your DB instead of building a string. Basically when you query your DB create an empty array and then when you loop the results of your DB to dynamically get the data use the javascript array.push method (or php equivalent if you're processing DB results in php) to push loop iteration data into a new array item. You should also use a console.log instead of an alert message so you do not need to close every alert message and essentially freeze your app while debugging.
OVER_QUERY_LIMIT is because you're trying to send too many geolocation requests (see 150 thousand explanation above), which is throttled by Google. You should be storing the geolocation result in your database so it doesn't attempt to geolocate a location that already has a geolocation saved in the DB. Also I'm not really sure why you're creating a var, trying to store in in localstorage, and then immediately retreiveing the local storage data to continue processing your markers. It seems redundant to do that, unless you're storing in local storage for some other reason. For debugging the marker issue it's best to strip out any unnecessary factors like the localstorage until you get it working with your markers and then refactor your localstorage logic into the script after a stripped down version is working.
best,
Shocker
Copy link to clipboard
Copied
Thanks so much for your help. Yes I see that using a string is wrong.
so you can see I set locations var at top.
- then you can see I attempted for add to the array with:
locations += ['[\'Location '+ cnt + ' Name\'', '\'' + value.getstr + ", " + value.getstate + '\'', '\'Location '+ cnt + ' URL\'], '];
i also tried this way:
newloc = '[\'Location '+ cnt + ' Name\'', '\'' + getstr + ", " + getcity + '\'', '\'Location '+ cnt + ' URL\'], ';
locations.push(newloc);
// got error with this as undefined
//Uncaught TypeError: Cannot read property 'push' of undefined
then use my locations var instead of hard coded - but it still has errors....
goal is to replace location = hard coded text with my db fields.
Q: I am probably adding to the array wrong? How Can I do this correctly?
my locations =
undefined['Location 1 Name','1410 N Lima St, Burbank','Location 1 URL'], ['Location 2 Name','3321 W Burbank Blvd, Burbank','Location 2 URL'], ['Location 3 Name','3321 W Burbank Blvd, Burbank','Location 3 URL'], ['Location 4 Name','3321 W Burbank Blvd, Burbank','Location 4 URL'], ['Location 5 Name','3321 W Burbank Blvd, Burbank','Location 5 URL'],
var locations = [];
var cnt = 1;
$.post('http://mysite.com/getaddresses.php', {
},
function(data, status){
console.log ('data', data);
output = "";
$.each(data, function(key, value) {
console. log ('item', key, value);
locations += ['[\'Location '+ cnt + ' Name\'', '\'' + value.getstr + ", " + value.getstate + '\'', '\'Location '+ cnt + ' URL\'], '];
cnt++;
});
// START map
//alert(locations2);
var locations = [
['Location 1 Name', 'New York, NY', 'Location 1 URL'],
['Location 2 Name', 'Newark, NJ', 'Location 2 URL'],
['Location 3 Name', 'Philadelphia, PA', 'Location 3 URL']
];
alert('from db '+locations);
var geocoder;
var map;
var bounds = new google.maps.LatLngBounds();
function initialize() {
map = new google.maps.Map(
document.getElementById("map_canvas"), {
center: new google.maps.LatLng(37.4419, -122.1419),
zoom: 13,
mapTypeId: google.maps.MapTypeId.ROADMAP
});
geocoder = new google.maps.Geocoder();
for (i = 0; i < locations.length; i++) {
geocodeAddress(locations, i);
}
}
google.maps.event.addDomListener(window, "load", initialize);
function geocodeAddress(locations, i) {
var title = locations[0];
var address = locations[1];
var url = locations[2];
geocoder.geocode({
'address': locations[1]
},
function (results, status) {
if (status == google.maps.GeocoderStatus.OK) {
var marker = new google.maps.Marker({
icon: 'http://maps.google.com/mapfiles/ms/icons/blue.png',
map: map,
position: results[0].geometry.location,
title: title,
animation: google.maps.Animation.DROP,
address: address,
url: url
})
infoWindow(marker, map, title, address, url);
bounds.extend(marker.getPosition());
map.fitBounds(bounds);
} else {
alert("geocode of " + address + " failed:" + status);
}
});
}
function infoWindow(marker, map, title, address, url) {
google.maps.event.addListener(marker, 'click', function () {
var html = "<div><h3>" + title + "</h3><p>" + address + "<br></div><a href='" + url + "'>View location</a></p></div>";
iw = new google.maps.InfoWindow({
content: html,
maxWidth: 350
});
iw.open(map, marker);
});
}
function createMarker(results) {
var marker = new google.maps.Marker({
icon: 'http://maps.google.com/mapfiles/ms/icons/blue.png',
map: map,
position: results[0].geometry.location,
title: title,
animation: google.maps.Animation.DROP,
address: address,
url: url
})
bounds.extend(marker.getPosition());
map.fitBounds(bounds);
infoWindow(marker, map, title, address, url);
return marker;
}
// END map
}); // END FUNCT
Copy link to clipboard
Copied
var locations = [];
$.post('http://xxxxxxxxxxx
//xxx start loop
$.each(data, function(key, value) {
newloc = '[\'Location '+ cnt + ' Name\'', '\'' + getstreet + ", " + getstate + '\'', '\'Location '+ cnt + ' URL\'], ';
locations.push(newloc);
cnt++;
//more xxxxxxxxxxxxxxx
my locations var fails as:
Uncaught TypeError: Cannot read property 'push' of undefined
console shows...
undefined['Location 1 Name','1410 N Lima St, CA','Location 1 URL'], ['Location 2 Name','3321 W Burbank Blvd, CA','Location 2 URL'], ['Location 3 Name','3558 W Burbank Blvd, CA','Location 3 URL'],
Q: How can I :
1 - fix the push error
2 - replace the hard coded working var locations with my version of locations and get it working?
Copy link to clipboard
Copied
It looks like I has a few errors
fix 1 ...
newloc = ['Location '+ cnt + ' Name\'', '\'' + getstr + ", " + gestate + '\'', '\'Location '+ cnt + ' URL\', '];
locations.push(newloc);
fix 2...
So it was fixed when I moved the location of this line (var locations) just BEFORE the .each loop like:
Code:
// old did not work var locations = [];
Q: does this NOT create array also - or is this bad formatting?
var locations = new Array();
$.each(data, function(key, value) { ...
//... but before, when It was higher (above the $.post('http://nmiapp. line ) it showed as undefined...
So i guess this is an issue of variable scope? Can you enlighten me how best to understand why it showed as undefined up higher?

