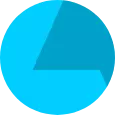
Copy link to clipboard
Copied
Hey guys, have a bit of an issue. Basically, I now have a third gallery which is different from the 2 I have been working on. I found an ideal component to use for this gallery. All the thumbnails have been set up perfectly using xml. Some of the thumbnails however will load an swf, and not images. At this moment, I have an xml like so
<images>
<image path="Work/work1/images/01.jpg"/>
<image path="Work/work1/images/02.jpg"/>
<image path="Work/work1/images/03.jpg"/>
<image path="Work/work1/images/04.jpg"/>
<image path="Work/work1/images/05.jpg"/>
<image path="Work/work1/images/06.jpg"/>
<image path="Work/work1/images/07.jpg"/>
<image path="Work/work1/images/08.swf"/>
<image path="Work/work1/images/09.swf"/>
<image path="Work/work1/images/10.swf"/>
</images>
Loading the big images is just a case of doing
function clickHandler1(event:ThumbnailEvent){
mySlideshow.loadImage(event.item.id);
}
I dont know how components are set up, but I have searched for the loadImage function and cannot find it anywhere. So what I thought I would do is try to add the swf's myself. I removed the swf links from the xml, and done this in my function
function clickHandler2(event:ThumbnailEvent){
var counter:Number = event.item.id + 4;
if(counter == 7){
myLoader1.load(new URLRequest("Work/work1/images/08.swf"));
mySlideshow.addChild(myLoader1);
trace("true1");
}
if(counter == 8){
myLoader2.load(new URLRequest("Work/work1/images/09.swf"));
mySlideshow.addChild(myLoader2);
trace("true2");
}
if (counter == 9) {
myLoader3.load(new URLRequest("Work/work1/images/10.swf"));
mySlideshow.addChild(myLoader3);
trace("true3");
}
else{
mySlideshow.loadImage(event.item.id + 4);
}
}
So what I am basically saying is if thumbnail 7, 8 or 9 is clicked, load their video, else load the image for the other clicked item. Now this somewhat works, but the first issue I faced is that if I clicked on a thumbnail and then another (thumbnail 7, 8 or 9), it would load one video on top of the other, and play both. So, before loading, I removedChild from mySlideshow where appropiate. This solved the issue of displaying the swf on top of the other, but the video contained within the swf that was removed would still be playing, as I can hear it
So I am not sure if firstly, I am approaching this the correct way, and secondly, how to stop the removed swf from playing.
Any advise appreciated as always
Nick
1 Correct answer
Update for the function:
function removeLoaders(loaders:Array):void {
for(var i:int = 0; i < loaders.length; i++) {
var loader:Loader = loaders as Loader;
if(!mySlideshow.contains(loader)) continue;
loader.unloadAndStop(true); mySlideshow.removeChild(loader); }}
Copy link to clipboard
Copied
Try this:
myLoader.unloadAndStop(true);
removeChild(myLoader);
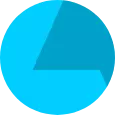
Copy link to clipboard
Copied
Now my solution to this is going to be a horrible one, because the only thing I could think of is doing something like this in each if statement
if(counter == 7){
if(mySlideshow.contains(myLoader2){
myLoader2.cancel(); //need to check if this is correct way, writing straight into post
myLoader2.unload();
mySlidshow.removeChild(myLoader2);
}if(mySlideshow.contains(myLoader3){
myLoader3.cancel();
myLoader3.unload();
mySlidshow.removeChild(myLoader3);
}
myLoader1.load(new URLRequest("Work/work1/images/08.swf"));
mySlideshow.addChild(myLoader1);
trace("true1");
}
I remember seeing before, I think you may have even shown me it, a way of doing something like this with a for loop instead. Is that possible or is the above the correct approach?
Copy link to clipboard
Copied
Update for the function:
function removeLoaders(loaders:Array):void {
for(var i:int = 0; i < loaders.length; i++) {
var loader:Loader = loaders as Loader;
if(!mySlideshow.contains(loader)) continue;
loader.unloadAndStop(true); mySlideshow.removeChild(loader); }}
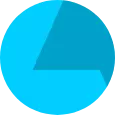
Copy link to clipboard
Copied
lol wicked, you are always one step ahead of me. I was just about to ask you if the previous function depended on the loaders having children. So what I would presume from this is that even if a child is removed from a movieclip, it still exists. The only real way to remove it and completely stop it from functioning would be to unloadAndStop?
Copy link to clipboard
Copied
Yes, removing from its parent means nothing. Ayny object to be removed from memory (garbage collected) has to have all connections severed.
Connections:
- existence in display list (removeChild(myObject)
- eventListeners (myObject.removeEventListener...)
- references, variables (myObject = null)
The Loader is more special because of its loading process.. I don't know how that does work actually, but you need to stop it too.
Copy link to clipboard
Copied
I see you have multiple loaders.. it would be better to create a function for it:
function removeLoaders(loaders:Array):void {
for(var i:int = 0; i < loaders.length; i++) {
var loader:Loader = loaders as Loader;
loader.unloadAndStop(true);
removeChild(loader);
}
}
Then use it:
function clickHandler2(event:ThumbnailEvent){ var counter:Number = event.item.id + 4; if(counter == 7){ myLoader1.load(new URLRequest("Work/work1/images/08.swf")); mySlideshow.addChild(myLoader1); removeLoaders([myLoader2, myLoader3]); trace("true1"); } if(counter == 8){ myLoader2.load(new URLRequest("Work/work1/images/09.swf")); mySlideshow.addChild(myLoader2);
removeLoaders([myLoader1, myLoader3]);
trace("true2"); } if (counter == 9) { myLoader3.load(new URLRequest("Work/work1/images/10.swf")); mySlideshow.addChild(myLoader3);
removeLoaders([myLoader1, myLoader2]);
trace("true3"); } else{ mySlideshow.loadImage(event.item.id + 4); }}

