is it possible to get a function pointer to a getter/setter function
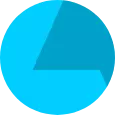
Copy link to clipboard
Copied
Please do not respond with questions asking why, what usage, or suggesting alternative ideas.
Looking for a yes/no answer, from someone who actually knows.
The generally used workaround for this, is to use a regular function instead of a getter/setter. This results in having to have ( ) after the function variable obviously. The question is, can a Function variable be assigned to a getter/setter function and retain its attribute of not needing brackets. Everything I have read seems to imply it is not, but I am looking for a definitive answer.
Below is some Pseudo code representing the question:
#########################################################
public class Foo(){
private var prop:Object;
public function get Prop():Object{return prop;}
public function set Prop(o:Object):void{prop=o;}
}
//==================
var GlobalFuncVar:Function = Foo.<get Prop>;
*** - If Foo.Prop is used, it returns the value. how can the function pointer be accessed...
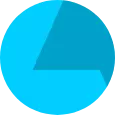
Copy link to clipboard
Copied
The answer Should be no, but with a dynamic Object you can make this posible where a property call is actually a shortcut to a function.
But this is a terrible practice because you will confuse anyone looking at the code when they feel its a property its actually a method being used.
NOT AN ALTERNATIVE SOLUTION, SAME SOLUTION WITH GOOD ENCAPSULATION, DELEGATE to an object.
use a get and set to set an object that has a common method to execute such as execute()
then call your prop functionCall or whatever you choose. myObject.functionCall= (ICanExecute type)
now that its in myObject.functionCall.ecex()
swap out your behavior that is required, but keep it clear to the user of your code.
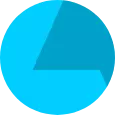
Copy link to clipboard
Copied
I am fully aware of the "terrible"ness of this, which is why I asked people not to ask regarding usage, alternatives etc. As I know people cannot help but respond in such ways. However, what you describe is part of the very issue.
"..because you will confuse anyone looking at the code when they feel its a property its actually a method being used."
^^^ This is quite close to the actual desired result in this case and why I simply want to know if its possible, since the a get/set is still just a function.
To be a little less vague, the point would be to replace a Global variable, with the property of a Global class. Such that it will be replaced with out needing to be retyped as either: VarName() or SomeClass.VarName but simply remain VarName, while actually be of type Function and pointing to the value of SomeClass.VarName.
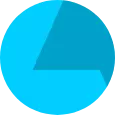
Copy link to clipboard
Copied
well so the asnwer you seek is yes, but i would still say that you should delegate.
public class yourClass{
var obj:Object
public function get foo():Object{
return obj.foo
}
public function set foo(obj:Object):void{
obj.foo=obj
}
}

