require some help making a random map.
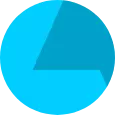
Copy link to clipboard
Copied
1) When you click on the "Generate" button a 15x21 board of Tile symbols is created, with top-left corner at (10,10). Initially all tiles should be blank.
i need to somehow get that done.
here is my code so far, i have a lot of graphics, movie clips and a button relating to the script, although right now they are not important.
var ghosts:Array = new Array();
var timers:Array = new Array();
//======== ghosts and ghost behaviour =================//
var ghostHoriz=1;
for (var row = 0; row < 4; row++) {
var g = new Ghost();
var t:Timer=new Timer(400);
g.timer=t;
timers[row]=t;
g.horiz=ghostHoriz;
ghostHoriz*=-1;
if (row==0||row==1) {
g.vert=1;
}
else {
g.vert=-1;
}
t.addEventListener(TimerEvent.TIMER, moveGhost);
ghosts[row]=g;
g.visible=false;
addChild(g);
}
var ghostIndex=0;
function moveGhost(evt:TimerEvent) {
var t=evt.currentTarget;
var k=timers.indexOf(t);
var g=ghosts
if (g.visible) {
var r=g.row;
var c=g.col;
with (g) {
if (Math.random()<0.5) {
if (((horiz > 0 && (c+horiz)<21)||(horiz < 0 && (c+horiz)>=0)) && (map
col+=horiz;
x+=horiz*20;
map
map
}
else {
horiz*=-1;
}
}
else {
if ( (( vert > 0 && (r+vert)<15)||( vert < 0 &&(r+vert)>= 0)) && (map[r+vert]
row+=vert;
y+=vert*20;
map[r+vert]
map
}
else {
vert*=-1;
}
}
}
}
}
// ================= Button behaviour ========== //
generate_btn.addEventListener(MouseEvent.CLICK, generate);
function generate(m:MouseEvent = null) {
initMap();
ghostIndex=0;
// other stuff
displayMap();
}
var map:Array = new Array();
var tiles:Array = new Array();
function initMap(){
}
function displayMap() {
for (var row = 0; row < 15; row++) {
for (var col = 0; col < 21; col++) {
var test=map[row][col];
tiles[row][col].x=10+col*20;
tiles[row][col].y=10+row*20;
switch (test) {
case 2 :
g=ghosts[ghostIndex];
g.x=10+col*20;
g.y=10+row*20;
g.row=row;
g.col=col;
if (row==0) {
g.vert=1;
}
else {
g.vert=-1;
}
g.visible=true;
g.timer.start();
ghostIndex++;
break;
}
}
}
}
bit long 😕
anyway when I basically need it so when you press generate it comes up with a random, how do i put this....it comes up with a random pacman sort of design. And everytime generate is clicked it changes, anyone know anything?
also note, with the code up there, if I do click generate i get this error...
Fonts should be embedded for any text that may be edited at runtime, other than text with the "Use Device Fonts" setting. Use the Text > Font Embedding command to embed fonts.
TypeError: Error #1010: A term is undefined and has no properties.
at Pacmanscripting_fla::MainTimeline/displayMap()
at Pacmanscripting_fla::MainTimeline/generate()
thanks in advance.
Copy link to clipboard
Copied
you have a 15x21 grid and 4 ghosts are to be assigned to 4 grid tiles?
Copy link to clipboard
Copied
Here is a conceptual blueprint on how to generate grid. Just paste code on timeline.
import flash.display.Sprite;
drawGrid();
function drawGrid():void
{
var rows:int = 15;
var cols:int = 21;
var numTiles:int = rows * cols;
for (var i:int = 0; i < numTiles; i++)
{
var t:Sprite = tile;
t.x = t.width * int(i / rows);
t.y = t.height * (i % rows);
addChild(t);
}
}
function get tile():Sprite
{
var t:Sprite = new Sprite();
t.graphics.beginFill(0xffffff * Math.random());
t.graphics.drawRect(0, 0, 30, 30);
return t;
}
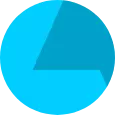
Copy link to clipboard
Copied
erm...i figured out where to past it, but once that happens the whole thing goes multicoloured and checkered....which is not really what I wanted.
mines supposed to end up like this:
https://shuspace.shu.ac.uk/courses/1/55-4885-00L-A-20123/content/_4655248_1/referralsoln_example.swf
if you have anything, please reply, but thank you so far !!!!
Copy link to clipboard
Copied
Your example requires login.
Copy link to clipboard
Copied
Here is how to do that from previously populated array - watch tiles:Array (still just a concept):
import flash.display.Sprite;
import flash.text.TextField;
import flash.text.TextFormat;
var tiles:Array;
var rows:int = 15;
var cols:int = 21;
getTiles();
drawGrid();
function getTiles():void
{
tiles = [];
var numTiles:int = rows * cols;
for (var i:int = 0; i < numTiles; i++)
{
tiles.push(drawTile(i));
}
}
function drawGrid():void
{
for (var i:int = 0; i < tiles.length; i++)
{
var t:Sprite = tiles;
t.x = t.width * int(i / rows);
t.y = t.height * (i % rows);
addChild(t);
}
}
function drawTile(index:int):Sprite
{
var tileSide:int = 40;
var color:uint = 0xffffff * Math.random();
var tile:Sprite = new Sprite();
tile.graphics.beginFill(color);
tile.graphics.drawRect(0, 0, tileSide, tileSide);
var label:TextField = new TextField();
label.defaultTextFormat = new TextFormat("Arial", 11, 0xffffff - color, "bold");
label.autoSize = "left";
label.text = String(index);
label.x = label.y = 4;
tile.addChild(label);
return tile;
}
Copy link to clipboard
Copied
This code is almost the same as a previous example but it randomizes tiles in the array - watch labels.
If you populate array with your tiles - you can be in business soon as far as the grid generation goes.
import flash.display.Sprite;
import flash.text.TextField;
import flash.text.TextFormat;
var tiles:Array;
var rows:int = 15;
var cols:int = 21;
getTiles();
drawGrid();
function getTiles():void
{
tiles = [];
var numTiles:int = rows * cols;
for (var i:int = 0; i < numTiles; i++)
{
tiles.push(drawTile(i));
}
shuffle(tiles);
}
function drawGrid():void
{
for (var i:int = 0; i < tiles.length; i++)
{
var t:Sprite = tiles;
t.x = t.width * int(i / rows);
t.y = t.height * (i % rows);
addChild(t);
}
}
function drawTile(index:int):Sprite
{
var tileSide:int = 40;
var color:uint = 0xffffff * Math.random();
var tile:Sprite = new Sprite();
tile.graphics.beginFill(color);
tile.graphics.drawRect(0, 0, tileSide, tileSide);
var label:TextField = new TextField();
label.defaultTextFormat = new TextFormat("Arial", 11, 0xffffff - color, "bold");
label.autoSize = "left";
label.text = String(index);
label.x = label.y = 4;
tile.addChild(label);
return tile;
}
function shuffle(a:Array):void
{
for (var i:int = a.length - 1; i >= 0; i--)
{
var r:int = Math.floor(Math.random() * (i + 1));
var t:Object = a
; a
= a; a = t;
}
}
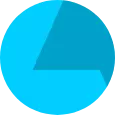
Copy link to clipboard
Copied
Hey this is really great so far, colour scheme is a little bright but i dont mind ! but i was wondering that if i am able to get the swf file, would you take a look at it and maybe help me out?
please ;-;...
Copy link to clipboard
Copied
Take a screenshot of how this puppy must look like and post it here.
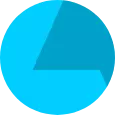
Copy link to clipboard
Copied
alright well this is the start screen. Once that generate button is clicked it spawns a grid, pacman stlye. i have more pics on the way.
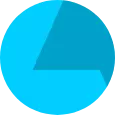
Copy link to clipboard
Copied
alright, once the generate button is clicked ,the above happens. Everytime generate is clicked the skulls which are the walls basically are changed positions randomley. The ghosts are still in their corner and pacman is still in the middle.
Copy link to clipboard
Copied
So, do you have two types of tiles - one with skull and another - without?
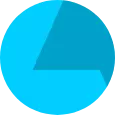
Copy link to clipboard
Copied
the tile actually has 2 keyframes inside of it....(is that how its worded) once double clicked you can see them, erm i dont mind sending you the file if you dont mind looking at it? just so we dont confuse things :3
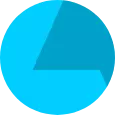
Copy link to clipboard
Copied
also the second keyframe inside the tile turns into a skull..
Copy link to clipboard
Copied
Post fla somewhere public (dropbox maybe) and I will look into it if I have time.
What is criteria for tile to go to the second frame? Is it supposed to happen randomly as well?
Copy link to clipboard
Copied
Also, how is tile linked in the library? What its class name?
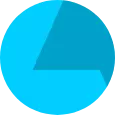
Copy link to clipboard
Copied
the tile is just called "Tile" if you click on it, it usually comes up with an image of it, it is a grey box with a thick white outline.
Copy link to clipboard
Copied
Try this. Replace entire code with this script. It generates one third of tiles with scull - 2/3 - without.
import flash.display.Sprite;
import flash.text.TextField;
import flash.text.TextFormat;
var tiles:Array;
var rows:int = 15;
var cols:int = 21;
var tilesContainer:Sprite;
init();
function init():void
{
generate_btn.addEventListener(MouseEvent.CLICK, drawGrid);
getTiles();
tilesContainer = new Sprite();
tilesContainer.x = tilesContainer.y = 10;
addChild(tilesContainer);
}
function getTiles():void
{
tiles = [];
var numTiles:int = rows * cols;
for (var i:int = 0; i < numTiles; i++)
{
var tile:Tile = new Tile();
tile.gotoAndStop((i % 3) == 0 ? 2 : 1);
tiles.push(tile);
}
}
function drawGrid(e:MouseEvent):void
{
shuffle(tiles);
for (var i:int = 0; i < tiles.length; i++)
{
var t:Tile = tiles;
t.x = t.width * int(i / rows);
t.y = t.height * (i % rows);
tilesContainer.addChild(t);
}
}
function shuffle(a:Array):void
{
for (var i:int = a.length - 1; i >= 0; i--)
{
var r:int = Math.floor(Math.random() * (i + 1));
var t:Object = a
; a
= a; a = t;
}
}
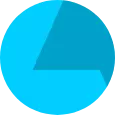
Copy link to clipboard
Copied
erm i also have this. Its basically a list of the things that need to be accomplished in this task D:
1) When you click on the "Generate" button a 15x21 board of Tile symbols is created, with top-left corner at (10,10). Initially all tiles should be blank.
2) Extend the functionality behind the "Generate" button so that a random configuration of walls appears - 100 of the tiles should now be "filled". Each time you click on "Generate" there should be a different configuration. Hint: create a map array initially consisting of 0's. Generate should randomly produce 100 pairs of (row, column) values where you set the value in the map array to 1. Use the map array to display the board.
3) Extend the "Generate" functionality even further. You should include in the application 4 instances of the Ghost symbol and one instance of the MyPac symbol. When you click on the "Generate" button, the ghosts should appear in each corner of the board, and the myPac should be in row 8, col 11 of the board. "Generate" should still produce a random configuration of 100 filled cells each time it is clicked, but the ghosts and myPac should always appear in the prescribed positions.
4) Finally, you should animate the ghosts and add key-handling for the myPac symbol. The ghosts should "patrol" the board ie they can move randomly in the array but cannot go through "walls" or myPac. The starting fla includes some code you might find useful for this part of the assignment. myPac should move with arrow-key presses, but also cannot go through walls or ghosts
if you are able to help me with steps 1-3 then thats enough. you dont need to do step 4 :3
Copy link to clipboard
Copied
There are too many requirements for a single thread.
You need to resolve issues one-by-one.
Let's assume this thread is devoted exclusively to creating grid. All other functionality I suggest you move to posts dedicated to to individual issue.
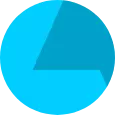
Copy link to clipboard
Copied
well you helped me with more than i could ask for, for that Im really thankful.... !!!!!
thank you again, meant a lot.
Copy link to clipboard
Copied
Mark this thread as answered and move to another one.
Are you sure that all grid creation issues are solved?
I suggest you play with this line:
tile.gotoAndStop((i % 3) == 0 ? 2 : 1);
Change it to
tile.gotoAndStop((i % 5) == 0 ? 2 : 1);
or
tile.gotoAndStop((i % 4) == 0 ? 2 : 1);
to find an optimal ratio of skull tiles.
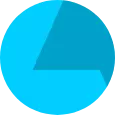
Copy link to clipboard
Copied
how do i mark this thread as answered? ._. new to this lol and yeah im sure that the grid problem is solved, works like a dream
Copy link to clipboard
Copied
Just mark the answer that you like as answered.

