Copy link to clipboard
Copied
What I am trying to do is take a string of numbers and convert it into an array, while cutting out the non-digits. Here is the format:
1.0000000e+000 1.3668423e+000
1.0000000e+000 1.3668423e+000
1.0000000e+000 1.3668423e+000
...
So it's basically: Space, Space, Digit, Space, Space, Digit, Newline
Right now I am using the following code (myString is a String, and dataSet1 is an Array):
var reg:RegExp = new RegExp("\n ");
myString = textLoader.data;
dataSet1 = myString.split(reg);
I've tried ("\n\s ") and ("(\n)(\s*)") and lots of other combinations, but I cannot seem to grasp how to do multiple conditions in a regular expression. All I need it to do is eliminate all spaces and newlines and I should be good.
I appreciate any and all help, thanks.
1 Correct answer

You could use the Array.map method to convert your strings. Something like this:
function str2Num(element:*, index:int, arr:Array):Number
{
return Number(element);
}
var arr:Array;
arr = myString.replace(/^\s+/,"").replace(/\s+$/,"").split(/\s+/);
arr = map(str2Num,null);
// or combine all in one statement
arr = myString.replace(/^\s+/,"").replace(/\s+$/,"").split(/\s+/).map(str2Num,null);
Copy link to clipboard
Copied
here ya go:
var reg:RegExp = /\n| /;
var arr:Array = myString.split(reg);
remember your array items will still be strings until you convert them to numbers
Copy link to clipboard
Copied
You are right, I need to convert my data into Nubmers (I think this is the appropriate data type). Is there a way for my to do a mass conversion on the Array, or can I do the conversion during the converstion from String to Array? Sorry, I know this isn't in the original question, but if you know how could you let me know, thanks!
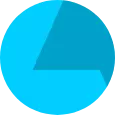
Copy link to clipboard
Copied
You could use the Array.map method to convert your strings. Something like this:
function str2Num(element:*, index:int, arr:Array):Number
{
return Number(element);
}
var arr:Array;
arr = myString.replace(/^\s+/,"").replace(/\s+$/,"").split(/\s+/);
arr = map(str2Num,null);
// or combine all in one statement
arr = myString.replace(/^\s+/,"").replace(/\s+$/,"").split(/\s+/).map(str2Num,null);
Copy link to clipboard
Copied
Thanks for all of the help. I believe I will be able to fix everything now.
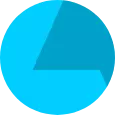
Copy link to clipboard
Copied
You're welcome.
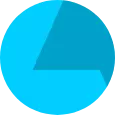
Copy link to clipboard
Copied
This removes both leading and trailing whitespace and then splits on any sequence of one or more whitespace characters:
var arr:Array = myString.replace(/^\s+/,"").replace(/\s+$/,"").split(/\s+/);
Copy link to clipboard
Copied
Thank you, this seems to work perfectly. But I have one last question or two. In the regular expression, what spaces are being replaced with the replaces method and then what spaces are left to be split? I also don't understand how this removes newline character? Here's the data format in the String (read from a file if it makes a difference):
[SPACE][SPACE or -][Number][SPACE][SPACE or -][Number][\n]
[SPACE][SPACE or -][Number][SPACE][SPACE or -][Number][\n]
[SPACE][SPACE or -][Number][SPACE][SPACE or -][Number][\n]
...etc
I guess that /^\s+/ removes the first one or two spaces, and /\s+$/ removes newline, and /\s+/ takes care of the inbetween spaces? I'm really not clear on this even with my book that discusses regular expressions, I just don't understands how it knows to target what parts.
Thanks.
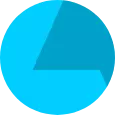
Copy link to clipboard
Copied
\s matches any whitespace character: space, tab, newline, return
/^\s+/ matches one or more whitespace characters at the beginning of the string
/\s+$/ matches one or more whitespace characters at the end of the string
var arr:Array = myString.replace(/^\s+/,"").replace(/\s+$/,"").split(/\s+/);
is the same as:
myString= myString.replace(/^\s+/,""); // remove leading whitespace
myString= myString.replace(/\s+$/,"") // remove trailing whitespace
var arr:Array= s.split(/\s+/); // split on remaining whitespace

