Checkbox Array
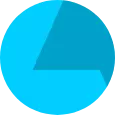
Copy link to clipboard
Copied
Hi
I am having a problem with a form/update database page.
I use a while loop to print a form for all the photos listed in a table - with their names/descriptions and the user must check a checkbox if he/she would like to include the photo in the main gallery. All goes OK except for my checkbox system.
I think the problem lies in that the checkbox array won't make a blank element if the checkbox has not been checked. ie. if I check the 20th and 30th photo's then the array will still think this to be the 1st and 2nd. I need to force the new array elements in the unchecked records so that on the update form I could use a conditional to set the values in the database. Can anyone see my problem? Thanks for any help. Chris
FORM:
while ($row = mysqli_fetch_array($r)) {
echo '<div class="photo-thumb">
<img src="../upload/' . rawurlencode($row['PhotoName']) . '" height="80px" />
<a href="delete_photo.php?id=' . $row['PhotoID'] . '&photoname=' . $row['PhotoName'] . '">Delete</a>
<p>' . $row['PhotoName'] . '</p>';
if ($row['Gallery'] == 1) {echo '<p>Photo Gallery: <input name="Gallery[]" id="Gallery" type="checkbox" value="1" checked="checked" /></p>';}
else {echo '<p>Photo Gallery: <input name="Gallery[]" id="Gallery" type="checkbox" value="1"/></p>';}
echo '<p>Description: <input name="PhotoDescription[]" id="PhotoDescription" type="text" maxlength="80" value="' . $row['PhotoDescription'] . '" /></p>
<input name="PhotoID[]" id="PhotoID" type="hidden" value="' . $row['PhotoID'] . '" />';
echo '</div>';
}
UPDATE PAGE:
<?php
$query = "SELECT * FROM photos";
$result = mysqli_query($dbc, $query);
$Total = count($_POST['PhotoID']);
for ($i = -1; $i <= $Total; $i++)
{
$PhotoDescription = $_POST['PhotoDescription'][$i];
$PhotoDescription = mysqli_real_escape_string($dbc, $PhotoDescription);
$PhotoID = $_POST['PhotoID'][$i];
if (!isset($_POST['Gallery'][$i])) {$Gallery = 0;}
else {$Gallery = 1;}
$q = "UPDATE photos SET PhotoDescription=\"$PhotoDescription\", Gallery='$Gallery' WHERE PhotoID=\"$PhotoID\"";
$r = mysqli_query($dbc, $q);
}
?>
Copy link to clipboard
Copied
I can see a couple of ways of handling this.
The simplest is to use a pair of radio buttons instead of a checkbox, and make the "don't include" option the default. As long as one radio button is always selected, the value will appear in the array.
The alternative would be to use a counter in the while loop in your form.
$thumb = 1;
while ($row = mysqli_fetch_array($r)) {
// inside the loop set the value of the checkbox to $thumb
// increment the counter at the end of the loop
$thumb++;
}
The value of each checkbox will then be a number. As you go through your update loop, you need to check the number:
if (isset($_POST['Gallery'][$i]) && $_POST['Gallery'] == $i + 1) {
$Gallery = 1;
} else {
$Gallery = 0;
}
Copy link to clipboard
Copied
Hang on. That second one won't work. I need to think about it a little more.
Copy link to clipboard
Copied
I think this should work:
if (isset($_POST['Gallery']) && in_array($i+1, $_POST['Gallery'])) {
$Gallery = 1;
} else {
$Gallery = 0;
}
This checks whether the Gallery array exists, and if it contains the current loop number + 1. You need to add the 1 because the while loop begins at 1.
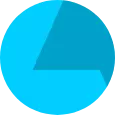
Copy link to clipboard
Copied
Hi David,
Thanks for your replies.
I'll give this a go and get back to you.
Many thanks,
Chris
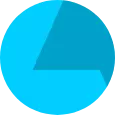
Copy link to clipboard
Copied
ahh still having a lot of problems. I'm a bit confused with your advice here though. I don't see how the $thumb variable changes anything with regard to the update page?
Sorry 😕 Thanks
Copy link to clipboard
Copied
The problem with checkbox groups is that a value is included in the $_POST array only if the checkbox is selected. So, setting the value of the checkbox to 1 to indicate that it has been selected is meaningless. You need to know which checkbox has been selected.
Using the $thumb variable as a counter in the loop will create a series of checkboxes each with a different value. The first will be 1, the second 2, the third 3, and so on.
You can then use the values in the $_POST['Gallery'] subarray to update your database. If $_POST['Gallery'] contains 5, 6, 7, 9, and 21, those are the records that need to add 1 to the database to indicate inclusion in the main gallery.
I started the $thumb counter at 1, but your update loop will begin at 0, so you need to check if $i+1 is in the $_POST['Gallery'] subarray.
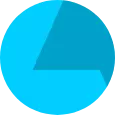
Copy link to clipboard
Copied
Thanks, David.
You have helped me a lot but I just managed to work out a slightly different way, though inspired by yours, that you might agree is simpler and I can't believe how I never thought of it before. We don't need to use the $thumb counter and then search the $thumb array. Instead I just did the same thing but with the photo's id!! How simple!
I put the value of the checkbox as the Photo's ID and on the update script's loop I just asked if the $PhotoID variable was in the $Gallery array. It works perfectly.
Thank you,
Chris
Copy link to clipboard
Copied
Sounds like a good idea. As long as you have a value to identify the selected item, that's what matters. Using an existing value is certainly better than creating another one.

