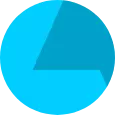
Copy link to clipboard
Copied
<?php virtual('/Transport/Connections/connect.php'); ?>
<?php
if (!function_exists("GetSQLValueString")) {
function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "")
{
if (PHP_VERSION < 6) {
$theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue;
}
$theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue);
switch ($theType) {
case "text":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "long":
case "int":
$theValue = ($theValue != "") ? intval($theValue) : "NULL";
break;
case "double":
$theValue = ($theValue != "") ? doubleval($theValue) : "NULL";
break;
case "date":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "defined":
$theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue;
break;
}
return $theValue;
}
}
$editFormAction = $_SERVER['PHP_SELF'];
if (isset($_SERVER['QUERY_STRING'])) {
$editFormAction .= "?" . htmlentities($_SERVER['QUERY_STRING']);
}
if ((isset($_POST["MM_insert"])) && ($_POST["MM_insert"] == "form1")) {
$insertSQL = sprintf("INSERT INTO bookingform (StuID, StuName, `Date`, `Time`, TransportType, Destination, NumPassenger, DepartVenue, NumberPhone, DriName, PlatNo, Status) VALUES (%s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s)",
GetSQLValueString($_POST['StuID'], "int"),
GetSQLValueString($_POST['StuName'], "text"),
GetSQLValueString($_POST['Date'], "text"),
GetSQLValueString($_POST['Time'], "text"),
GetSQLValueString($_POST['TransportType'], "text"),
GetSQLValueString($_POST['Destination'], "text"),
GetSQLValueString($_POST['NumPassenger'], "text"),
GetSQLValueString($_POST['DepartVenue'], "text"),
GetSQLValueString($_POST['NumberPhone'], "int"),
GetSQLValueString($_POST['DriName'], "text"),
GetSQLValueString($_POST['PlatNo'], "text"),
GetSQLValueString($_POST['Status'], "text"));
mysql_select_db($database_connect, $connect);
$Result1 = mysql_query($insertSQL, $connect) or die(mysql_error());
$insertGoTo = "MenuStudent.php";
if (isset($_SERVER['QUERY_STRING'])) {
$insertGoTo .= (strpos($insertGoTo, '?')) ? "&" : "?";
$insertGoTo .= $_SERVER['QUERY_STRING'];
}
header(sprintf("Location: %s", $insertGoTo));
}
mysql_select_db($database_connect, $connect);
$query_BookAdd = "SELECT * FROM bookingform";
$BookAdd = mysql_query($query_BookAdd, $connect) or die(mysql_error());
$row_BookAdd = mysql_fetch_assoc($BookAdd);
$totalRows_BookAdd = mysql_num_rows($BookAdd);
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html lang="en">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Untitled Document</title>
</head>
<body>
<h1><center> Booking Form </center></h1>
<center>
<p>Fill the following details in the booking form </p>
<form action="<?php echo $editFormAction; ?>" method="post" name="form1" id="form1">
<table align="center">
<tr valign="baseline">
<td width="134" align="right" nowrap="nowrap">Student ID:</td>
<td width="192"><input type="text" name="StuID" value="" size="32" /></td>
</tr>
<tr valign="baseline">
<td nowrap="nowrap" align="right">Student Name:</td>
<td><input type="text" name="StuName" value="" size="32" /></td>
</tr>
<tr valign="baseline">
<td nowrap="nowrap" align="right">Date:</td>
<td><input type="text" name="Date" value="YYYY/MM/DD" size="32" /></td>
</tr>
<tr valign="baseline">
<td nowrap="nowrap" align="right">Time:</td>
<td><input type="text" name="Time" value="HH:MM" size="32" /></td>
</tr>
<tr valign="baseline">
<td nowrap="nowrap" align="right">Type of transport:</td>
<td><label for="TransportType"></label>
<select name="TransportType" id="TransportType">
<option value = "Bus"> Bus</option>
<option value = "Van" >Van</option>
<option value = "Car" >Car</option>
</select></td>
</tr>
<tr valign="baseline">
<td nowrap="nowrap" align="right">Destination:</td>
<td><input type="text" name="Destination" value="" size="32" /></td>
</tr>
<td nowrap="nowrap" align="right">Number of Passenger:</td>
<td><label for="NumPasseger"></label>
<select name="NumPasseger" id="NumPasseger">
<option value ="1 - 5" >1-5</option>
<option value = "5 - 10" >5-10</option>
<option value = "10 - 15" >10-15</option>
<option value = "15 - 20" >15-20</option>
</select></td>
<tr valign="baseline">
<td nowrap="nowrap" align="right">Departure Venue :</td>
<td><label for="DepartVenue"></label>
<select name="DepartVenue" id="DepartVenue">
<option value = "K1">K1</option>
<option value = "K2">K2</option>
</select></td>
</tr>
<tr valign="baseline">
<td nowrap="nowrap" align="right">Phone Number:</td>
<td><input type="text" name="NumberPhone" value="" size="32" /></td>
</tr>
<tr valign="baseline">
<td colspan="2" align="center" nowrap="nowrap">
<input type="submit" value="Submit Form" /></td>
</tr>
</table>
<p> </p>
<form>
<a href="MenuStudent.php">Main Menu</a>
</form>
</form>
<form id="CancelReport" name="CancelReport" method="post" action="MenuStudent.php">
<input type="submit" name="Cancel" id="Cancel" value="Cancel" />
</form>
<p> </p>
<p> </p>
</center>
</p>
</body>
</html>
<?php
mysql_free_result($BookData);
?>
I tried
1 Correct answer
The code you're using is outdated. You should not be using deprecated Dreamweaver Server-Behaviors code to create modern web applications. The connection methods are not secure and more importantly, they won't work on a server running PHP 7 or higher. In fact, you will have problems with PHP 5.6 if deprecated error reporting is not disabled.
Modern developers use MySQLi (improved) or PDO for CRUD pages (create, read, update & delete data records).
Nancy
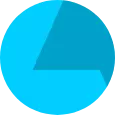
Copy link to clipboard
Copied
Tried using this code, the value does not entered in the database. Help me out if there's any error with the php codes tq
Copy link to clipboard
Copied
The code you're using is outdated. You should not be using deprecated Dreamweaver Server-Behaviors code to create modern web applications. The connection methods are not secure and more importantly, they won't work on a server running PHP 7 or higher. In fact, you will have problems with PHP 5.6 if deprecated error reporting is not disabled.
Modern developers use MySQLi (improved) or PDO for CRUD pages (create, read, update & delete data records).
Nancy
Copy link to clipboard
Copied
Check what version of php your local or remote server is running. As Nancy says the code you are using is beyond its sell by date and will not work if you are using a version of php 7+ - eliminate that possibility first by checking the version.

