How to find list of conditional tags in a book using script?
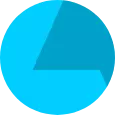
Copy link to clipboard
Copied
How to find list of conditional tags in a book using script?
[Thread moved to scripting forum by moderator]
Copy link to clipboard
Copied
Hi, here is a quick ExtendScript that should do this. Files must be open to be processed. It is loosely tested, uncommented, and has no guarantees. Hope this helps.
Russ
if (typeof Array.prototype.indexOf != "function")
{
Array.prototype.indexOf = function (val, startIndex)
{
if(startIndex == null) startIndex = 0;
for(var i = startIndex; i < this.length; i++)
{
if(val === this) return i;
}
return -1;
}
}
ListConditionTagsInBook(app.ActiveBook);
function ListConditionTagsInBook(book)
{
if(book == null || !book.ObjectValid()) book = app.ActiveBook;
if(!book.ObjectValid()) book = app.FirstOpenBook;
if(!book.ObjectValid())
{
alert("No active book. Cannot continue.");
return;
}
var conditionNames = new Array(0);
var filesProcessed = 0;
var filesSkipped = 0;
var comp = book.FirstComponentInBook;
while(comp.ObjectValid())
{
if(comp.ComponentType == Constants.FV_BK_FILE)
{
doc = FindOpenDoc(comp.Name);
if(doc != null)
{
var conditionObj = doc.FirstCondFmtInDoc;
while(conditionObj.ObjectValid())
{
if(conditionNames.indexOf(conditionObj.Name) < 0)
conditionNames.push(conditionObj.Name);
conditionObj = conditionObj.NextCondFmtInDoc;
} //end condition while
filesProcessed++;
} // end doc if
else
filesSkipped++;
} // end component type if
comp = comp.NextBookComponentInDFSOrder;
} //end component while
var msg = "Operation completed. Files processed: "
+ filesProcessed
+ ". Files skipped because they were closed: "
+ filesSkipped
+ ".\n\nConditions found ("
+ conditionNames.length
+ " total):\n\n";
for(var i = 0; i < conditionNames.length; i++)
msg += conditionNames + "\n";
alert(msg);
}
//Send a fully qualified path. Returns null if the doc
//is not open in the session.
function FindOpenDoc(path)
{
var doc= null;
var filePath, comparePath;
doc = app.FirstOpenDoc;
while(doc.ObjectValid())
{
if(doc.Name.toLowerCase() == path.toLowerCase())
break;
else doc = doc.NextOpenDocInSession;
}
if(!doc.ObjectValid()) doc = null;
return doc;
}

