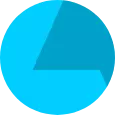
Copy link to clipboard
Copied
Hello, i am not a math wonder and am stuck with generating a sequence of numbers.
I have an array of sprites where i want to loop through and set it's x position.
But i really want to do this with a loop, but i dont know to calculate these sequence of number (5,4,3,2,1,0, 11, 10, 9, 8, 7, 6, 17, 16, 15,14,13,12)
Without the loop it looks like: (What i am doing is setting the first sprite xposition to the last one in that row and then the second sprite to the lastone minus 1 and so one..) Does anyone know a way to do this through math?
myArray[0].x = myArray[5].origX;
myArray[1].x = myArray[4].origX;
myArray[2].x = myArray[3].origX;
myArray[3].x = myArray[2].origX;
myArray[4].x = myArray[1].origX;
myArray[5].x = myArray[0].origX;
myArray[6].x = myArray[11].origX;
myArray[7].x = myArray[10].origX;
myArray[8].x = myArray[9].origX;
myArray[9].x = myArray[8].origX;
myArray[10].x = myArray[7].origX;
myArray[11].x = myArray[6].origX;
myArray[12].x = myArray[17].origX;
myArray[13].x = myArray[16].origX;
myArray[14].x = myArray[16].origX;
myArray[15].x = myArray[14].origX;
myArray[16].x = myArray[13].origX;
myArray[17].x = myArray[12].origX;
1 Correct answer
The most efficient way to calculate column based grid it like this:
// number of columns
var numCols:int = 6;
var column:int = 0;
var row:int = 0;
for (var i:int = 0; i < 18; i++) {
column = i % numCols;
row = int(i / numCols);
trace(i, "\tcolumn =", column, "row =", row);
}
As for your swap, one of abstract ways to do that can be:
...// number of columns
var numCols:int = 6;
var column:int = 0;
var row:int = 0;
// alter ego of index
var swap:int = 0;
for (var i:int = 0; i < 18; i++) {
column = i
Copy link to clipboard
Copied
for(var i:uint=0;i<3;i++)
{
for(var ii:uint=0;ii<6;ii++)
{
var num:int=(i+1)*6-(ii+1);
trace(num);
}
}
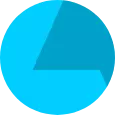
Copy link to clipboard
Copied
Thanks, you example does the trick, but i was wondering if it could be done
something like this, so within one loop:
var rowcounter:int =1;
var counter:int = 0;
for(var i:int = 0 ; i<17; i++) {
var num:int = i%6;
trace(num, rowcounter);
if(num == 5) {
rowcounter++;
}
//counter = 17/i*rowCounter ..something?
}
Like could i now calculate the counter value like the number sequence in my previous post?
Copy link to clipboard
Copied
The most efficient way to calculate column based grid it like this:
// number of columns
var numCols:int = 6;
var column:int = 0;
var row:int = 0;
for (var i:int = 0; i < 18; i++) {
column = i % numCols;
row = int(i / numCols);
trace(i, "\tcolumn =", column, "row =", row);
}
As for your swap, one of abstract ways to do that can be:
// number of columns
var numCols:int = 6;
var column:int = 0;
var row:int = 0;
// alter ego of index
var swap:int = 0;
for (var i:int = 0; i < 18; i++) {
column = i % cols;
swap = numCols + i - column * 2 - 1;
trace(i, "=>", swap);
}
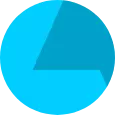
Copy link to clipboard
Copied
Thanks! That is exactly what i was looking for! points well earned!
I only needed to rename the cols to numCols in you example and it worked!
I think this way is better for performance then to use more loops

