How edit a running script and replace some variables
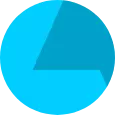
Copy link to clipboard
Copied
var RedFirst = 0;
var GreenFirst = 0;
var BlueFirst = 0;
var RedSecond = 1;
var GreenSecond = 1;
var BlueSecond = 1;
var RedFirstCopy = RedFirst;
var GreenFirstCopy = GreenFirst;
var BlueFirstCopy = BlueFirst;
var RedSecondCopy = RedSecond;
var GreenSecondCopy = GreenSecond;
var BlueSecondCopy = BlueSecond;
var MainPanel = new Window ("dialog", "Main Panel");
var Text1 = MainPanel.add ("statictext");
Text1.text = "First color";
var RSlider1 = MainPanel.add ("slider");
RSlider1.minvalue = 0;
RSlider1.maxvalue = 255;
RSlider1.value = Math.round (RedFirstCopy * 255);
var RNumber1 = MainPanel.add ("statictext", undefined, RSlider1.value);
RNumber1.preferredSize.width = 25;
RNumber1.graphics.foregroundColor = RNumber1.graphics.newPen (RNumber1.graphics.PenType.SOLID_COLOR, [1, 0, 0], 1);
RNumber1.graphics.disabledForegroundColor = RNumber1.graphics.foregroundColor;
RNumber1.graphics.font = ScriptUI.newFont (RNumber1.graphics.font.name, "Bold", RNumber1.graphics.font.size);
var GSlider1 = MainPanel.add ("slider");
GSlider1.minvalue = 0;
GSlider1.maxvalue = 255;
GSlider1.value = Math.round (GreenFirstCopy * 255);
var GNumber1 = MainPanel.add ("statictext", undefined, GSlider1.value);
GNumber1.preferredSize.width = 25;
GNumber1.graphics.foregroundColor = GNumber1.graphics.newPen (GNumber1.graphics.PenType.SOLID_COLOR, [0, 1, 0], 1);
GNumber1.graphics.disabledForegroundColor = GNumber1.graphics.foregroundColor;
GNumber1.graphics.font = ScriptUI.newFont (GNumber1.graphics.font.name, "Bold", GNumber1.graphics.font.size);
var BSlider1 = MainPanel.add ("slider");
BSlider1.minvalue = 0;
BSlider1.maxvalue = 255;
BSlider1.value = Math.round (BlueFirstCopy * 255);
var BNumber1 = MainPanel.add ("statictext", undefined, BSlider1.value);
BNumber1.preferredSize.width = 25;
BNumber1.graphics.foregroundColor = BNumber1.graphics.newPen (BNumber1.graphics.PenType.SOLID_COLOR, [0, 0, 1], 1);
BNumber1.graphics.disabledForegroundColor = BNumber1.graphics.foregroundColor;
BNumber1.graphics.font = ScriptUI.newFont (BNumber1.graphics.font.name, "Bold", BNumber1.graphics.font.size);
RSlider1.onChanging = GSlider1.onChanging = BSlider1.onChanging = function () {
RNumber1.text = Math.round (RSlider1.value);
GNumber1.text = Math.round (GSlider1.value);
BNumber1.text = Math.round (BSlider1.value);
RedFirstCopy = Math.floor ((Math.round (RSlider1.value) / 255) * 100) / 100;
GreenFirstCopy = Math.floor ((Math.round (GSlider1.value) / 255) * 100) / 100;
BlueFirstCopy = Math.floor ((Math.round (BSlider1.value) / 255) * 100) / 100;
}
var Text2 = MainPanel.add ("statictext");
Text2.text = "Second color";
var RSlider2 = MainPanel.add ("slider");
RSlider2.minvalue = 0;
RSlider2.maxvalue = 255;
RSlider2.value = Math.round (RedSecondCopy * 255);
var RNumber2 = MainPanel.add ("statictext", undefined, RSlider2.value);
RNumber2.preferredSize.width = 25;
RNumber2.graphics.foregroundColor = RNumber2.graphics.newPen (RNumber2.graphics.PenType.SOLID_COLOR, [1, 0, 0], 1);
RNumber2.graphics.disabledForegroundColor = RNumber2.graphics.foregroundColor;
RNumber2.graphics.font = ScriptUI.newFont (RNumber2.graphics.font.name, "Bold", RNumber2.graphics.font.size);
var GSlider2 = MainPanel.add ("slider");
GSlider2.minvalue = 0;
GSlider2.maxvalue = 255;
GSlider2.value = Math.round (GreenSecondCopy * 255);
var GNumber2 = MainPanel.add ("statictext", undefined, GSlider2.value);
GNumber2.preferredSize.width = 25;
GNumber2.graphics.foregroundColor = GNumber2.graphics.newPen (GNumber2.graphics.PenType.SOLID_COLOR, [0, 1, 0], 1);
GNumber2.graphics.disabledForegroundColor = GNumber2.graphics.foregroundColor;
GNumber2.graphics.font = ScriptUI.newFont (GNumber2.graphics.font.name, "Bold", GNumber2.graphics.font.size);
var BSlider2 = MainPanel.add ("slider");
BSlider2.minvalue = 0;
BSlider2.maxvalue = 255;
BSlider2.value = Math.round (BlueSecondCopy * 255);
var BNumber2 = MainPanel.add ("statictext", undefined, BSlider2.value);
BNumber2.preferredSize.width = 25;
BNumber2.graphics.foregroundColor = BNumber2.graphics.newPen (BNumber2.graphics.PenType.SOLID_COLOR, [0, 0, 1], 1);
BNumber2.graphics.disabledForegroundColor = BNumber2.graphics.foregroundColor;
BNumber2.graphics.font = ScriptUI.newFont (BNumber2.graphics.font.name, "Bold", BNumber2.graphics.font.size);
RSlider2.onChanging = GSlider2.onChanging = BSlider2.onChanging = function () {
RNumber2.text = Math.round (RSlider2.value);
GNumber2.text = Math.round (GSlider2.value);
BNumber2.text = Math.round (BSlider2.value);
RedSecondCopy = Math.floor ((Math.round (RSlider2.value) / 255) * 100) / 100;
GreenSecondCopy = Math.floor ((Math.round (GSlider2.value) / 255) * 100) / 100;
BlueSecondCopy = Math.floor ((Math.round (BSlider2.value) / 255) * 100) / 100;
}
var Apply = MainPanel.add ("button", undefined, "Apply changes");
Apply.onClick = function () {
var JSX = File ($.fileName);
JSX.open ("r");
var JSXXX = JSX.read ();
JSX.close ();
JSX.open ("w");
JSXXX = JSXXX.replace (RedFirst, RedFirstCopy);
JSXXX = JSXXX.replace (GreenFirst, GreenFirstCopy);
JSXXX = JSXXX.replace (BlueFirst, BlueFirstCopy);
JSXXX = JSXXX.replace (RedSecond, RedSecondCopy);
JSXXX = JSXXX.replace (GreenSecond, GreenSecondCopy);
JSXXX = JSXXX.replace (BlueSecond, BlueSecondCopy);
JSX.write(JSXXX);
JSX.close ();
Pannello1.close ();
$.gc ();
(Script = new ActionDescriptor).putPath (stringIDToTypeID ("javaScript"), new File ($.fileName));
Script.putString (stringIDToTypeID ("javaScriptMessage"), "0");
executeAction (stringIDToTypeID ("AdobeScriptAutomation Scripts"), Script, DialogModes.NO);
}
MainPanel.show ();
Explore related tutorials & articles
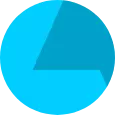
Copy link to clipboard
Copied
Sorry, I made a mistake, the normal colors are used for coloring the panel, while the copy are a placeholder used to store the changes.
The normal colors should be overwritten by the placeholders.
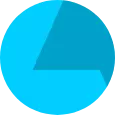
Copy link to clipboard
Copied
Since apparently nobody is able to solve this problem, I opted for this method:
Copy link to clipboard
Copied
The problem with your original code was that with the replace function you were only looking for a value, not caring which line it was found on.
For example, the initial value of RedFirst = 0; You change it to 0.64 with the replace(0, 0.64) function. Ok, for the first tine it worked.
The original value of GreenFirst = 0, you change it to 0.5 using the replace(0, 0.5) function, but this time the replace function will find the number zero not in the line you expect, but in RedFirst = 0.64 (you see zero there, right? So replace function also sees it), as a result you will get GreenFirst = 0.5.64
That is, you had to write in the replace function not only the value, but the text from the string with the variable, allowing you to uniquely identify it.
By the way, @Ghoul Fool gave a good example of how to get data using XML object, but writing data in your example by reference looks terrible. It can be simplified as follows:
var RedFirst = 0;
var GreenFirst = 0;
var BlueFirst = 0;
var RedSecond = 1;
var GreenSecond = 1;
var BlueSecond = 1;
var myXML= new XML("<variables></variables>")
myXML.RedFirst = RedFirst;
myXML.GreenFirst = GreenFirst;
myXML.BlueFirst = BlueFirst;
myXML.RedSecond = RedSecond;
myXML.GreenSecond = GreenSecond;
myXML.BlueSecond = BlueSecond;
var XMLFile = File (Folder.desktop + "/config.xml");
XMLFile.encoding = "UTF8"
XMLFile.open ('w');
XMLFile.write(myXML.toXMLString())
XMLFile.close ();
Copy link to clipboard
Copied
Adobe has a sample script in the ESTK distribution that does something similar. Maybe look at that.
Copy link to clipboard
Copied
var settings = new File(app.preferencesFolder + "/{e8960d2f-a19d-4941-9355-6b136bede99e}.dat");
if (settings.exists) $.evalFile(settings);
var RedFirst;
var GreenFirst;
var BlueFirst;
var RedSecond;
var GreenSecond;
var BlueSecond;
if (RedFirst == undefined) RedFirst = 0;
if (GreenFirst == undefined) GreenFirst = 0;
if (BlueFirst == undefined) BlueFirst = 0;
if (RedSecond == undefined) RedSecond = 1;
if (GreenSecond == undefined) GreenSecond = 1;
if (BlueSecond == undefined) BlueSecond = 1;
/*
....
*/
function save_settings()
{
try {
settings.open("w");
settings.writeln("var RedFirst = " + RedFirstCopy);
settings.writeln("var GreenFirst = " + GreenFirstCopy);
settings.writeln("var BlueFirst = " + BlueFirstCopy);
settings.writeln("var RedSecond = " + RedSecondCopy);
settings.writeln("var GreenSecond = " + GreenSecondCopy);
settings.writeln("var BlueSecond = " + BlueSecondCopy);
if (settings.error) { alert("Params save error!", "Error", true); settings.close(); return false; }
settings.close();
return true;
}
catch (e) { alert(e); }
}

