Copy link to clipboard
Copied
Hi!
I'm now working on a forward mapping effect.
For every pixel, I sample the luma of another layer, use it as a Z value, compute 3D displacement, sample the source layer and fill output with the color (using PF_FILL).
I guess it can be improved, so here are my questions:
1_what is the best way to store coordinates, and data in general? I first thought a PF_EffectWorld would do the trick, but in 8bits, values are limited to 255...
2_is PF_FILL the most efficient way to paint a pixel? As I use forward mapping, I don't fill output in the same order than I scan the input, and I don't know how to fill a specific pixel directly.
3_Does anyone know a good way to interpolate the empty pixels after forward mapping? For the moment, I just fill "bigger" pixels according to distance from camera...
Seems using Kernels could be a solution, but I have no idea how to use it...
That's already a lot of questions... If you have any clue, it'll be really appreciated!
Thanx,
François
1 Correct answer

1. Why store the pixel data of the world if you got access to both layers' pixel data which you can access directly? Or did I misunderstand you? Of course you can also make your own PF_EffectWorld (or any other memory structure) and copy the pixels to that, but that obviously won't increase the resolution.
2. There are examples in the SDK and also in the manual on how to access pixels directly. I use the following functions myself:
inline PF_PixelFloat* getPixel(PF_EffectWorld* inputP, const A_lon
...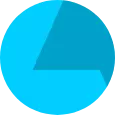
Copy link to clipboard
Copied
1. Why store the pixel data of the world if you got access to both layers' pixel data which you can access directly? Or did I misunderstand you? Of course you can also make your own PF_EffectWorld (or any other memory structure) and copy the pixels to that, but that obviously won't increase the resolution.
2. There are examples in the SDK and also in the manual on how to access pixels directly. I use the following functions myself:
inline PF_PixelFloat* getPixel(PF_EffectWorld* inputP, const A_long x, const A_long y) { return (PF_PixelFloat*)((char*)inputP->data + (y * inputP->rowbytes) + x * sizeof(PF_PixelFloat)); };
inline void setPixel(PF_EffectWorld* inputP, const A_long x, const A_long y, const PF_PixelFloat* col) { *((PF_PixelFloat*)((char*)inputP->data + (y * inputP->rowbytes) + x * sizeof(PF_PixelFloat))) = *col; };
Keep in mind: these do not perform boundary checking and are only suitable for PF_PixelFloat - for other bitdepths, simply replace the struct or make a template.
3. Not quite sure what you mean here. If you want to use kernels, you can either use Adobe's own functions (as presented in one of the SDk samples I think), but in my opinion you are better off with just writing your own kernel loops, as you have more control then.
Copy link to clipboard
Copied
Hi Toby,
Thanx for your answers.
1.I need to store the data so I can compute the pixels in a different order than the "scan lines" (for example, sort them by Z value), and interpolate them. I'm not using any library or ready made function.
2.Thanx for the function! That's exactly what I was looking for. As I don't have programer background, I'm a bit lost with memory handling and all my attempts to do the same finished with crash...
3.I didn't find any good example of kernel use. But I probably misunderstood kernel's use too... So I 'll follow your advice and try to do it myself.
Thanx again!
Cheers,
François
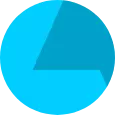
Copy link to clipboard
Copied
Using the above functions you can quickly set and get a pixel value of any layer at any time without the need to use scanlines. You can also use the PF_SAMPLINGSUITE to do nearest neighbour or interpolated sampling using the SDK. I think the shifter example uses subsampling of a layer.
Copy link to clipboard
Copied
Yes, the functions you kindly showed are really what I needed. In the sample plugins, the samplesuites are mostly used inside scanline loops.
Thanx again!
François
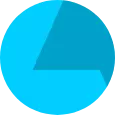
Copy link to clipboard
Copied
It is probably a good idea to use a safe version of these function that check if the coordinates are within bounds, else you might overwrite memory you are not supposed to.
Adding something along "if (x >= 0 && x < inputP->width && y >= 0 && y < inputP->height) ..." will do the trick.
Also adapting it to your specific needs (ie. not returning a pointer to the data but modifying inplace) could make sense.
As I said, you can also use the sampling suite to get the value of any pixel in any layer, with the added bonus of interplation.
Code would be something like that (out of my head):
static PF_Pixel samplePixel(PF_InData* in_data, PF_EffectWorld* inputP, const A_FpShort x, const A_FpShort y)
{
AEGP_SuiteHandler suites(in_data->pica_basicP);
PF_Pixel col;
PF_SampPB samp_pb; samp_pb.src = inputP;
suites.Sampling8Suite1()->subpixel_sample(in_data->effect_ref, FLOAT_2_FIX(x), FLOAT_2_FIX(y), &samp_pb, &col);
return col;
};
Copy link to clipboard
Copied
I already added the "if (x >= 0 && x < inputP->width && y >= 0 && y < inputP->height)" condition 🙂
For the subpixelSampling function, it does interpolate, but I think I need to write my own interpolation, as I need to find values for missing data (pixels are spread out by forward mapping, unlike reverse mapping). That's also why I need to store data.
I think I wasn't really clear on this point in my first post...
Thanx again for this really complete answer!
Cheers,
François

